注釈
クリック here 完全なサンプルコードをダウンロードします
新しい座標類(射手座用)を作成する¶
本稿では,後述するように,定義球面座標フレームのサブクラスをどのように分割して定義するかを詳細に紹介する. 新しい枠組みを定義する 和の文書文字列 BaseCoordinateFrame
それがそうです。この例では、Majewskiらが定義したように、人馬座低銀河軌道平面によって定義される座標系(以下、SGRと略す)を定義する。2003年)。SGR座標系は通常2つの角座標を指します \(\Lambda,B\) それがそうです。
そのためには BaseCoordinateFrame
これは,各サポートの表現における座標系角度の名前と単位を知っている.この場合私たちは SphericalRepresentation
“Lambda”と“Beta”を使ってそしてこの座標系から他の内蔵座標系への変換を定義しなければならない.銀河座標を使って Galactic
級友たち。
別項参照¶
♪the gala package 恒星流座標系には多くのAstropy座標系が定義されている。
Majewskiら。2003年、“射手座低銀河2ミクロン終日巡回天図。I.射手座コアと潮汐腕の形態学”、https://arxiv.org/abs/estio-ph/0304198
Law&Majewski,2010年,“人馬座低銀河:銀河三軸ハローの進化モデル”,https://arxiv.org/abs/1003.1132
David LawのSgr情報ページhttps://www.stsor.edu/~dlaw/sgr/
By: Adrian Price-Whelan, Erik Tollerud
ライセンス:BSD
製造 print
すべてのバージョンのPythonで同じ方法を使用して、numpy、matplotlibを設定し、より良い描画パラメータのセットを使用します:
import numpy as np
import matplotlib.pyplot as plt
from astropy.visualization import astropy_mpl_style
plt.style.use(astropy_mpl_style)
座標を導入するために必要なバッグ
from astropy.coordinates import frame_transform_graph
from astropy.coordinates.matrix_utilities import rotation_matrix, matrix_product, matrix_transpose
import astropy.coordinates as coord
import astropy.units as u
最初のステップは新しいクラスを作ることです Sagittarius
それを可能にしました BaseCoordinateFrame
:
class Sagittarius(coord.BaseCoordinateFrame):
"""
A Heliocentric spherical coordinate system defined by the orbit
of the Sagittarius dwarf galaxy, as described in
https://ui.adsabs.harvard.edu/abs/2003ApJ...599.1082M
and further explained in
https://www.stsci.edu/~dlaw/Sgr/.
Parameters
----------
representation : `~astropy.coordinates.BaseRepresentation` or None
A representation object or None to have no data (or use the other keywords)
Lambda : `~astropy.coordinates.Angle`, optional, must be keyword
The longitude-like angle corresponding to Sagittarius' orbit.
Beta : `~astropy.coordinates.Angle`, optional, must be keyword
The latitude-like angle corresponding to Sagittarius' orbit.
distance : `Quantity`, optional, must be keyword
The Distance for this object along the line-of-sight.
pm_Lambda_cosBeta : :class:`~astropy.units.Quantity`, optional, must be keyword
The proper motion along the stream in ``Lambda`` (including the
``cos(Beta)`` factor) for this object (``pm_Beta`` must also be given).
pm_Beta : :class:`~astropy.units.Quantity`, optional, must be keyword
The proper motion in Declination for this object (``pm_ra_cosdec`` must
also be given).
radial_velocity : :class:`~astropy.units.Quantity`, optional, must be keyword
The radial velocity of this object.
"""
default_representation = coord.SphericalRepresentation
default_differential = coord.SphericalCosLatDifferential
frame_specific_representation_info = {
coord.SphericalRepresentation: [
coord.RepresentationMapping('lon', 'Lambda'),
coord.RepresentationMapping('lat', 'Beta'),
coord.RepresentationMapping('distance', 'distance')]
}
プログレッシブ分解ではこのようなものを BaseCoordinateFrame
それがそうです。そして,記述文書文字列を含む.最後の行はクラスクラス属性であり,データのデフォルト表示形式,速度情報のデフォルト差分,およびオブジェクトが使用する属性名から使用する名前へのマッピングを指定する. Sagittarius
フレームワーク。この場合、球形表現における名前をカバーするが、デカルト表現または円柱表現のような他の表現は使用されない。
次に,この座標系から他の内蔵座標系への変換を定義しなければならず,銀河座標系を用いる.これは,変換行列を返す関数を定義したり,座標を受け取り,新しいシステムで新しい座標を返す関数を簡単に定義することで実現できる.射手座座標系への変換は銀河座標からの球面回転のみであるため,1つの関数のみを定義してこの行列を返す.私たちはあらかじめ決められたオイラー角と rotation_matrix
ヘルパー関数:
SGR_PHI = (180 + 3.75) * u.degree # Euler angles (from Law & Majewski 2010)
SGR_THETA = (90 - 13.46) * u.degree
SGR_PSI = (180 + 14.111534) * u.degree
# Generate the rotation matrix using the x-convention (see Goldstein)
D = rotation_matrix(SGR_PHI, "z")
C = rotation_matrix(SGR_THETA, "x")
B = rotation_matrix(SGR_PSI, "z")
A = np.diag([1.,1.,-1.])
SGR_MATRIX = matrix_product(A, B, C, D)
上の変換(回転)行列を構築しており,回転行列の逆はその転置であるため,必要な変換関数は非常に簡単である.
@frame_transform_graph.transform(coord.StaticMatrixTransform, coord.Galactic, Sagittarius)
def galactic_to_sgr():
""" Compute the transformation matrix from Galactic spherical to
heliocentric Sgr coordinates.
"""
return SGR_MATRIX
装飾師. @frame_transform_graph.transform(coord.StaticMatrixTransform, coord.Galactic, Sagittarius)
この関数を frame_transform_graph
座標変換とする.関数内部では,先に定義した回転行列のみを返す.
そして、回転行列の転置を用いて逆変換を登録する(逆変換よりも計算速度が速い)。
@frame_transform_graph.transform(coord.StaticMatrixTransform, Sagittarius, coord.Galactic)
def sgr_to_galactic():
""" Compute the transformation matrix from heliocentric Sgr coordinates to
spherical Galactic.
"""
return matrix_transpose(SGR_MATRIX)
私たちはこれらの変換を登録しました Sagittarius
そして Galactic
私たちは any 座標系と Sagittarius
(別のシステムに変換する経路があれば Galactic
)である。たとえば,ICRS座標を変換する. Sagittarius
私たちはそうします
icrs = coord.SkyCoord(280.161732*u.degree, 11.91934*u.degree, frame='icrs')
sgr = icrs.transform_to(Sagittarius)
print(sgr)
出て:
<SkyCoord (Sagittarius): (Lambda, Beta) in deg
(346.81830652, -39.28360407)>
あるいは、私たちが Sagittarius
フレームからICRS座標まで(本例では、エッジである Sagittarius
X-y平面):
sgr = coord.SkyCoord(Lambda=np.linspace(0, 2*np.pi, 128)*u.radian,
Beta=np.zeros(128)*u.radian, frame='sagittarius')
icrs = sgr.transform_to(coord.ICRS)
print(icrs)
出て:
<SkyCoord (ICRS): (ra, dec) in deg
[(284.03876751, -29.00408353), (287.24685769, -29.44848352),
(290.48068369, -29.81535572), (293.7357366 , -30.1029631 ),
(297.00711066, -30.30991693), (300.28958688, -30.43520293),
(303.57772919, -30.47820084), (306.86598944, -30.43869669),
(310.14881715, -30.31688708), (313.42076929, -30.11337526),
(316.67661568, -29.82915917), (319.91143548, -29.46561215),
(323.12070147, -29.02445708), (326.30034928, -28.50773532),
(329.44683007, -27.9177717 ), (332.55714589, -27.257137 ),
(335.62886847, -26.52860943), (338.66014233, -25.73513624),
(341.64967439, -24.87979679), (344.59671212, -23.96576781),
(347.50101283, -22.99629167), (350.36280652, -21.97464811),
(353.18275454, -20.90412969), (355.96190618, -19.78802107),
(358.70165491, -18.62958199), ( 1.40369557, -17.43203397),
( 4.06998374, -16.19855028), ( 6.70269788, -14.93224899),
( 9.30420479, -13.63618882), ( 11.87702861, -12.31336727),
( 14.42382347, -10.96672102), ( 16.94734952, -9.59912794),
( 19.45045241, -8.21341071), ( 21.93604568, -6.81234162),
( 24.40709589, -5.39864845), ( 26.86661004, -3.97502106),
( 29.31762493, -2.54411871), ( 31.76319801, -1.10857781),
( 34.20639942, 0.32898001), ( 36.65030466, 1.76593955),
( 39.09798768, 3.19968374), ( 41.55251374, 4.6275852 ),
( 44.01693189, 6.04699804), ( 46.49426651, 7.45524993),
( 48.98750752, 8.84963453), ( 51.4995989 , 10.22740448),
( 54.03342512, 11.58576509), ( 56.59179508, 12.92186896),
( 59.17742314, 14.23281165), ( 61.79290712, 15.51562883),
( 64.44070278, 16.76729487), ( 67.12309478, 17.98472356),
( 69.84216409, 19.16477088), ( 72.59975183, 20.30424045),
( 75.39742013, 21.3998918 ), ( 78.23641033, 22.44845192),
( 81.11759966, 23.44663022), ( 84.04145735, 24.39113719),
( 87.00800203, 25.27870692), ( 90.01676196, 26.10612335),
( 93.06674057, 26.87025019), ( 96.15638947, 27.56806406),
( 99.28359159, 28.19669038), (102.44565666, 28.75344107),
(105.63933131, 29.23585315), (108.86082534, 29.64172698),
(112.105855 , 29.96916281), (115.36970341, 30.21659414),
(118.64729687, 30.38281659), (121.93329519, 30.46701088),
(125.22219273, 30.46875885), (128.50842634, 30.38805179),
(131.78648572, 30.22529063), (135.05102157, 29.98127794),
(138.29694697, 29.6572022 ), (141.51952827, 29.2546151 ),
(144.71446203, 28.77540295), (147.87793614, 28.22175338),
(151.00667382, 27.59611901), (154.09796066, 26.90117914),
(157.14965528, 26.13980125), (160.16018547, 25.31500315),
(163.12853176, 24.42991703), (166.05420084, 23.48775622),
(168.93719133, 22.49178507), (171.77795423, 21.44529257),
(174.57735037, 20.35156967), (177.33660656, 19.21389046),
(180.05727218, 18.03549704), (182.74117737, 16.81958784),
(185.39039367, 15.56930924), (188.00719783, 14.28774998),
(190.59403895, 12.97793826), (193.15350938, 11.64284103),
(195.68831902, 10.28536518), (198.20127316, 8.90836046),
(200.69525342, 7.51462369), (203.17320154, 6.10690412),
(205.63810576, 4.6879097 ), (208.09298919, 3.26031403),
(210.54090002, 1.82676397), (212.984903 , 0.38988751),
(215.42807182, -1.04769799), (217.87348209, -2.48337744),
(220.32420429, -3.91452965), (222.7832966 , -5.338519 ),
(225.25379684, -6.75268736), (227.73871349, -8.15434631),
(230.24101506, -9.54076983), (232.76361762, -10.90918763),
(235.30937003, -12.25677927), (237.88103647, -13.58066929),
(240.48127601, -14.87792359), (243.11261883, -16.14554723),
(245.777439 , -17.38048408), (248.47792364, -18.57961852),
(251.2160385 , -19.7397795 ), (253.9934903 , -20.85774736),
(256.81168612, -21.93026371), (259.67169071, -22.95404466),
(262.57418275, -23.92579758), (265.51941137, -24.84224172),
(268.50715471, -25.70013256), (271.53668252, -26.49628998),
(274.6067251 , -27.22762983), (277.71545113, -27.89119849),
(280.86045662, -28.48420985), (284.03876751, -29.00408353)]>
一例として、2つの座標系の点をプロットします。
fig, axes = plt.subplots(2, 1, figsize=(8, 10),
subplot_kw={'projection': 'aitoff'})
axes[0].set_title("Sagittarius")
axes[0].plot(sgr.Lambda.wrap_at(180*u.deg).radian, sgr.Beta.radian,
linestyle='none', marker='.')
axes[1].set_title("ICRS")
axes[1].plot(icrs.ra.wrap_at(180*u.deg).radian, icrs.dec.radian,
linestyle='none', marker='.')
plt.show()
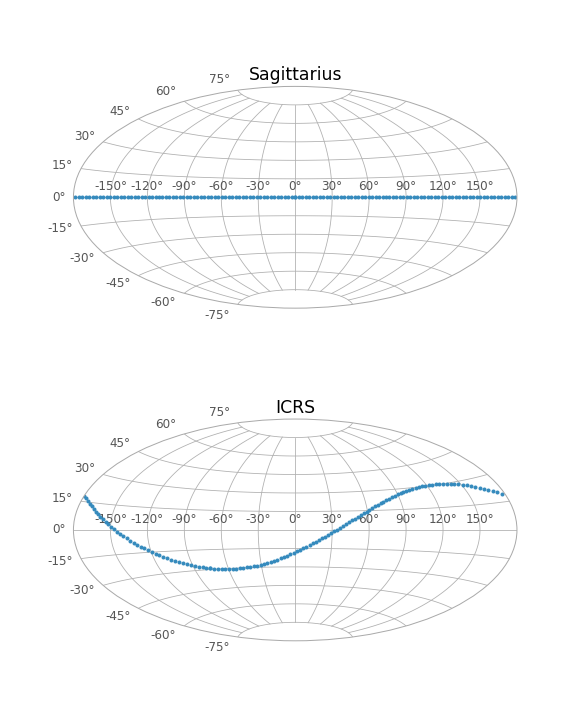
この特殊な変換は1つの球面回転のみであり,これはベクトルオフセットのないアフィン変換の特例である.したがって,速度成分の変換も自機によってサポートされる:
sgr = coord.SkyCoord(Lambda=np.linspace(0, 2*np.pi, 128)*u.radian,
Beta=np.zeros(128)*u.radian,
pm_Lambda_cosBeta=np.random.uniform(-5, 5, 128)*u.mas/u.yr,
pm_Beta=np.zeros(128)*u.mas/u.yr,
frame='sagittarius')
icrs = sgr.transform_to(coord.ICRS)
print(icrs)
fig, axes = plt.subplots(3, 1, figsize=(8, 10), sharex=True)
axes[0].set_title("Sagittarius")
axes[0].plot(sgr.Lambda.degree,
sgr.pm_Lambda_cosBeta.value,
linestyle='none', marker='.')
axes[0].set_xlabel(r"$\Lambda$ [deg]")
axes[0].set_ylabel(
fr"$\mu_\Lambda \, \cos B$ [{sgr.pm_Lambda_cosBeta.unit.to_string('latex_inline')}]")
axes[1].set_title("ICRS")
axes[1].plot(icrs.ra.degree, icrs.pm_ra_cosdec.value,
linestyle='none', marker='.')
axes[1].set_ylabel(
fr"$\mu_\alpha \, \cos\delta$ [{icrs.pm_ra_cosdec.unit.to_string('latex_inline')}]")
axes[2].set_title("ICRS")
axes[2].plot(icrs.ra.degree, icrs.pm_dec.value,
linestyle='none', marker='.')
axes[2].set_xlabel("RA [deg]")
axes[2].set_ylabel(
fr"$\mu_\delta$ [{icrs.pm_dec.unit.to_string('latex_inline')}]")
plt.show()
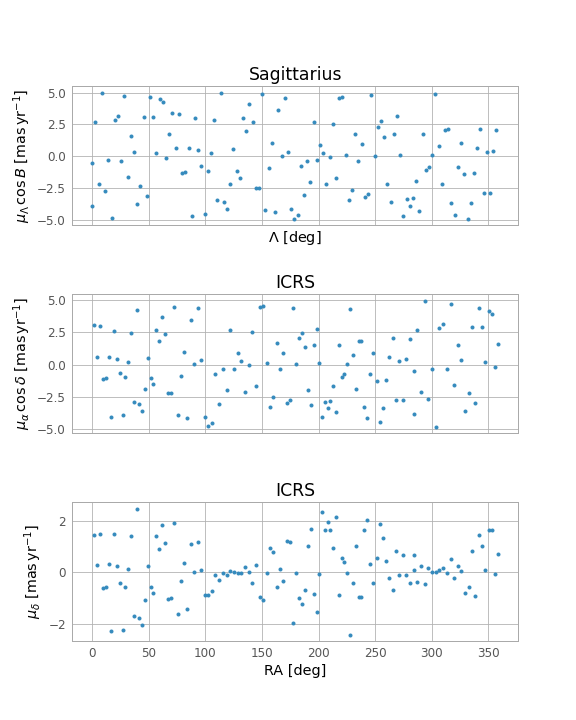
出て:
<SkyCoord (ICRS): (ra, dec) in deg
[(284.03876751, -29.00408353), (287.24685769, -29.44848352),
(290.48068369, -29.81535572), (293.7357366 , -30.1029631 ),
(297.00711066, -30.30991693), (300.28958688, -30.43520293),
(303.57772919, -30.47820084), (306.86598944, -30.43869669),
(310.14881715, -30.31688708), (313.42076929, -30.11337526),
(316.67661568, -29.82915917), (319.91143548, -29.46561215),
(323.12070147, -29.02445708), (326.30034928, -28.50773532),
(329.44683007, -27.9177717 ), (332.55714589, -27.257137 ),
(335.62886847, -26.52860943), (338.66014233, -25.73513624),
(341.64967439, -24.87979679), (344.59671212, -23.96576781),
(347.50101283, -22.99629167), (350.36280652, -21.97464811),
(353.18275454, -20.90412969), (355.96190618, -19.78802107),
(358.70165491, -18.62958199), ( 1.40369557, -17.43203397),
( 4.06998374, -16.19855028), ( 6.70269788, -14.93224899),
( 9.30420479, -13.63618882), ( 11.87702861, -12.31336727),
( 14.42382347, -10.96672102), ( 16.94734952, -9.59912794),
( 19.45045241, -8.21341071), ( 21.93604568, -6.81234162),
( 24.40709589, -5.39864845), ( 26.86661004, -3.97502106),
( 29.31762493, -2.54411871), ( 31.76319801, -1.10857781),
( 34.20639942, 0.32898001), ( 36.65030466, 1.76593955),
( 39.09798768, 3.19968374), ( 41.55251374, 4.6275852 ),
( 44.01693189, 6.04699804), ( 46.49426651, 7.45524993),
( 48.98750752, 8.84963453), ( 51.4995989 , 10.22740448),
( 54.03342512, 11.58576509), ( 56.59179508, 12.92186896),
( 59.17742314, 14.23281165), ( 61.79290712, 15.51562883),
( 64.44070278, 16.76729487), ( 67.12309478, 17.98472356),
( 69.84216409, 19.16477088), ( 72.59975183, 20.30424045),
( 75.39742013, 21.3998918 ), ( 78.23641033, 22.44845192),
( 81.11759966, 23.44663022), ( 84.04145735, 24.39113719),
( 87.00800203, 25.27870692), ( 90.01676196, 26.10612335),
( 93.06674057, 26.87025019), ( 96.15638947, 27.56806406),
( 99.28359159, 28.19669038), (102.44565666, 28.75344107),
(105.63933131, 29.23585315), (108.86082534, 29.64172698),
(112.105855 , 29.96916281), (115.36970341, 30.21659414),
(118.64729687, 30.38281659), (121.93329519, 30.46701088),
(125.22219273, 30.46875885), (128.50842634, 30.38805179),
(131.78648572, 30.22529063), (135.05102157, 29.98127794),
(138.29694697, 29.6572022 ), (141.51952827, 29.2546151 ),
(144.71446203, 28.77540295), (147.87793614, 28.22175338),
(151.00667382, 27.59611901), (154.09796066, 26.90117914),
(157.14965528, 26.13980125), (160.16018547, 25.31500315),
(163.12853176, 24.42991703), (166.05420084, 23.48775622),
(168.93719133, 22.49178507), (171.77795423, 21.44529257),
(174.57735037, 20.35156967), (177.33660656, 19.21389046),
(180.05727218, 18.03549704), (182.74117737, 16.81958784),
(185.39039367, 15.56930924), (188.00719783, 14.28774998),
(190.59403895, 12.97793826), (193.15350938, 11.64284103),
(195.68831902, 10.28536518), (198.20127316, 8.90836046),
(200.69525342, 7.51462369), (203.17320154, 6.10690412),
(205.63810576, 4.6879097 ), (208.09298919, 3.26031403),
(210.54090002, 1.82676397), (212.984903 , 0.38988751),
(215.42807182, -1.04769799), (217.87348209, -2.48337744),
(220.32420429, -3.91452965), (222.7832966 , -5.338519 ),
(225.25379684, -6.75268736), (227.73871349, -8.15434631),
(230.24101506, -9.54076983), (232.76361762, -10.90918763),
(235.30937003, -12.25677927), (237.88103647, -13.58066929),
(240.48127601, -14.87792359), (243.11261883, -16.14554723),
(245.777439 , -17.38048408), (248.47792364, -18.57961852),
(251.2160385 , -19.7397795 ), (253.9934903 , -20.85774736),
(256.81168612, -21.93026371), (259.67169071, -22.95404466),
(262.57418275, -23.92579758), (265.51941137, -24.84224172),
(268.50715471, -25.70013256), (271.53668252, -26.49628998),
(274.6067251 , -27.22762983), (277.71545113, -27.89119849),
(280.86045662, -28.48420985), (284.03876751, -29.00408353)]
(pm_ra_cosdec, pm_dec) in mas / yr
[(-0.50379352, 0.08702402), ( 2.6953726 , -0.39002803),
(-2.11353031, 0.24583331), ( 4.96538766, -0.4351699 ),
(-2.6679841 , 0.15675154), (-0.30208592, 0.00897834),
(-4.80805404, 0.00296548), ( 2.8201224 , 0.0803428 ),
( 3.14395899, 0.18085715), (-0.35088406, -0.03032364),
( 4.70529711, 0.54160133), (-1.564708 , -0.22454628),
( 1.55696736, 0.26711001), ( 0.36150435, 0.07200772),
(-3.60524306, -0.81598496), (-2.21033699, -0.5590445 ),
( 2.94580445, 0.82156629), (-2.97449063, -0.90478693),
( 4.40088648, 1.44668672), ( 2.92152276, 1.02973692),
( 0.21697034, 0.08143828), ( 4.14412712, 1.64646766),
( 3.9495738 , 1.65205822), (-0.15099074, -0.06617175),
( 1.56212564, 0.71411101), ( 3.04333686, 1.44531738),
( 0.60419905, 0.29697674), ( 2.97163773, 1.50640586),
(-1.15192063, -0.60025865), (-1.04449598, -0.55774464),
( 0.58223531, 0.31764922), (-4.08732313, -2.27180504),
( 2.64376531, 1.49295021), ( 0.42869514, 0.24530484),
(-0.68160971, -0.39418803), (-3.88161969, -2.26302501),
(-0.97733008, -0.57298419), ( 0.23992634, 0.14110183),
( 2.42467531, 1.42691021), (-2.9123389 , -1.71084133),
( 4.26155814, 2.49283549), (-3.05595666, -1.77563329),
(-3.5856898 , -2.06428001), (-1.89662661, -1.07908608),
( 0.48268772, 0.27069539), (-1.0107912 , -0.55724358),
(-1.48237889, -0.80112392), ( 2.68583499, 1.41878045),
( 1.79482719, 0.92391811), ( 3.69731189, 1.84876332),
( 2.39269415, 1.1582249 ), (-2.20742971, -1.03069502),
(-2.22906539, -1.00002977), ( 4.50677706, 1.93451143),
(-3.91959148, -1.60235902), (-0.87039388, -0.3371644 ),
( 0.99060632, 0.36155567), (-4.15613873, -1.42017477),
( 3.4544351 , 1.09709488), ( 0.06117723, 0.01790645),
( 4.41062057, 1.17802995), ( 0.35243612, 0.08487621),
(-4.06546188, -0.86990998), (-4.79487895, -0.89472692),
(-4.50222652, -0.71481275), (-0.72010219, -0.09400818),
(-3.03655975, -0.30975514), (-0.36618876, -0.02681202),
(-2.00650481, -0.08878887), ( 2.67049236, 0.040521 ),
(-0.30599155, 0.00426566), ( 0.87433901, -0.03761438),
( 0.26585303, -0.01914009), (-2.15830278, 0.21754284),
(-0.03023846, 0.0039112 ), ( 2.49119684, -0.3925642 ),
(-1.63537253, 0.30324748), ( 4.47593422, -0.95259556),
( 4.52378022, -1.08435526), ( 0.0932935 , -0.0248151 ),
(-3.29032292, 0.95954746), (-2.50509695, 0.792991 ),
( 1.67861316, -0.57190392), (-0.32665829, 0.11890875),
( 0.88766022, -0.34302776), (-2.96313329, 1.20871949),
(-2.74131773, 1.17438083), ( 4.37558506, -1.95952914),
( 0.01926368, -0.00898013), ( 2.04996984, -0.99088284),
( 2.48536667, -1.24113588), ( 1.35671936, -0.69758181),
(-1.94318508, 1.02541986), (-3.1171945 , 1.68310317),
( 1.55639797, -0.85735961), ( 2.76073099, -1.54720515),
( 0.08858496, -0.05037237), (-4.07278051, 2.34364932),
(-2.87318772, 1.66886978), (-3.35745418, 1.96351195),
(-2.83989103, 1.66806563), (-1.63745503, 0.96360973),
(-3.69048577, 2.1705612 ), ( 1.51765842, -0.88992706),
(-0.94563277, 0.55147204), (-0.70807458, 0.40965429),
( 0.0611101 , -0.03498554), ( 4.2814047 , -2.41921728),
( 0.71176419, -0.39589866), (-1.86908214, 1.02057477),
( 1.82086238, -0.9732545 ), ( 1.86640365, -0.97363928),
(-3.25793649, 1.6535767 ), (-4.12342369, 2.02954392),
(-0.72597622, 0.34530303), ( 0.93414157, -0.42775881),
(-1.27590729, 0.56021613), (-4.48513078, 1.87996301),
(-3.37354304, 1.34338065), (-1.19341705, 0.44907351),
( 0.59809989, -0.21140015), ( 2.03992516, -0.67266261),
(-2.72845699, 0.83282735), ( 0.31812168, -0.0890675 ),
(-2.75097141, 0.69884582), ( 0.43806271, -0.09964658),
( 2.02196952, -0.40510038), (-3.83008647, 0.66159945)]>
スクリプトの総実行時間: (0分0.445秒)