注釈
クリック here 完全なサンプルコードをダウンロードします
3色画像(JPG)を分離適合画像に変換する¶
この例は、RGB JPEG画像を開き、各チャネルを別個のFITS(画像)ファイルとして書き込む。
この例で使用する pillow 画像を読み取るためには matplotlib.pyplot
画像を表示し astropy.io.fits
FITSファイルを保存する場合は、以下の操作を実行してください。
By: Erik Bray, Adrian Price-Whelan
ライセンス:BSD
import numpy as np
from PIL import Image
from astropy.io import fits
Matplotlibを設定し、より良い描画パラメータのセットを使用する
import matplotlib.pyplot as plt
from astropy.visualization import astropy_mpl_style
plt.style.use(astropy_mpl_style)
元の3色jpeg画像をロードして表示します:
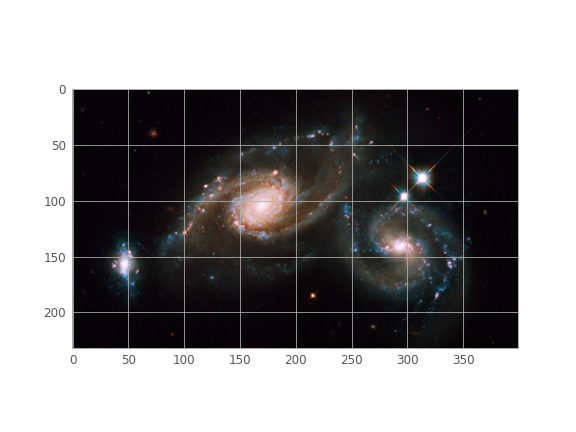
出て:
Image size: 232 x 400
Image bands: ('R', 'G', 'B')
3つのチャネル(RGB)を分割し、Numpy配列の形態でデータを取得する。配列はフラットなので1次元です
出て:
(92800,)
画像配列を2次元に再構成する:
出て:
(232, 400)
チャネルを単独の適合画像として書き込む.タイトル情報の追加と可視化
red = fits.PrimaryHDU(data=r_data)
red.header['LATOBS'] = "32:11:56" # add spurious header info
red.header['LONGOBS'] = "110:56"
red.writeto('red.fits')
green = fits.PrimaryHDU(data=g_data)
green.header['LATOBS'] = "32:11:56"
green.header['LONGOBS'] = "110:56"
green.writeto('green.fits')
blue = fits.PrimaryHDU(data=b_data)
blue.header['LATOBS'] = "32:11:56"
blue.header['LONGOBS'] = "110:56"
blue.writeto('blue.fits')
from pprint import pprint
pprint(red.header)
出て:
SIMPLE = T / conforms to FITS standard
BITPIX = 64 / array data type
NAXIS = 2 / number of array dimensions
NAXIS1 = 400
NAXIS2 = 232
EXTEND = T
LATOBS = '32:11:56'
LONGOBS = '110:56 '
作成したファイルを削除する
スクリプトの総実行時間: (0分0.195秒)